Remote MCP Server
Tools are managed by the workspace admins. They can add, and remove tools from the workspace, and assign them to specific space.
If you want to add a specific remote server to the workspace, contact your workspace admin, you'll need to give them the URL of the server you want to add. With it, they'll be able to follow this guide to add the MCP server to the workspace.
Remote MCP Servers and how to add them.
As an administrator, you can add a Remote MCP Server to your workspace. To do so, go to the Tools section of the Knowledge Tab. Here, you'll find an Add Tool button.
Clicking this Add Tool button will open a list of Dust-built MCP Servers, and a button to add some Remote MCP Servers.
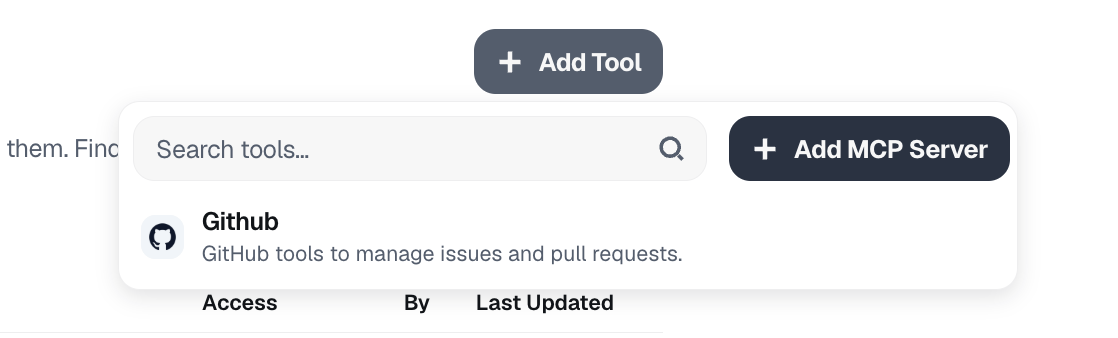
The interface to add an MCP server
Remote MCP Servers are not managed by Dust, which means that you can use any MCP Server available on the internet. Plenty of MCP providers exists out there and are usable out of the box with Dust.
Some providers handle authentication themselves, by authenticating the server with one account. This means that using them in Dust will do all the calls with the account authenticated to the server. One way to circumvent it is to create one server per person, and to add them to some restricted spaces. This way, the authentication for a person will only be usable inside their own restricted space.
You can also create a MCP Server yourself, host it in your infrastructure and plug it directly into Dust.
On the click of the Add MCP Server button, you'll only have to enter your server's URL, and after a quick synchronization, your server will be created and ready to be added, as a toolset, to your agents.
Remote MCP Servers Available by Default
The following remote MCP servers are available by default for the admin to configure without reference the server's URL:
Customize your Remote MCP Server.
Servers are customizable. You can change their name, and their description. Those are not exposed to the model and are only for UI/UX purposes, to help member of your workspaces distinguish the toolsets they have access to.
MCP Servers can give a lot of different capabilities to your agents, and we feel like your users needs to realize what is happening under the hood. That's why we implemented a "Stake" level for every tool, of each toolset. Two levels exists :
- The "High" stake, meaning that the tool may update data or send information. This is the default stake.
- The "Low" stake, meaning that the tool will only retrieve data or generate content.
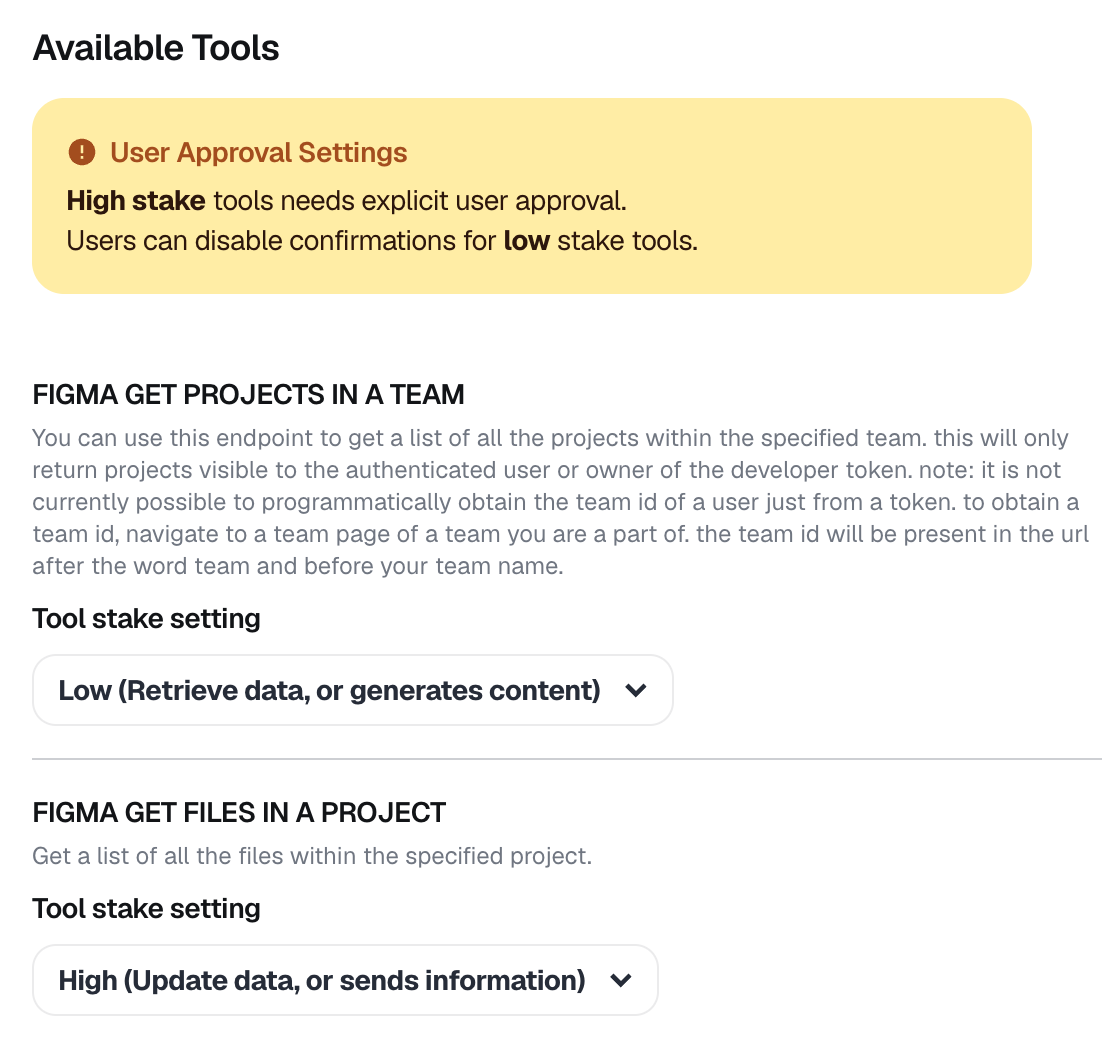
A list of tools, with drop-downs to select the stake of each action
A High stake action will always trigger a validation pop-up when the tool is used. The user will need to acknowledge the use of the tool by the agent. A Low stake action can be silenced by the user at their own will. Once they silence the tool, they won't see the validation pop-ups anymore, and the action will execute automatically.
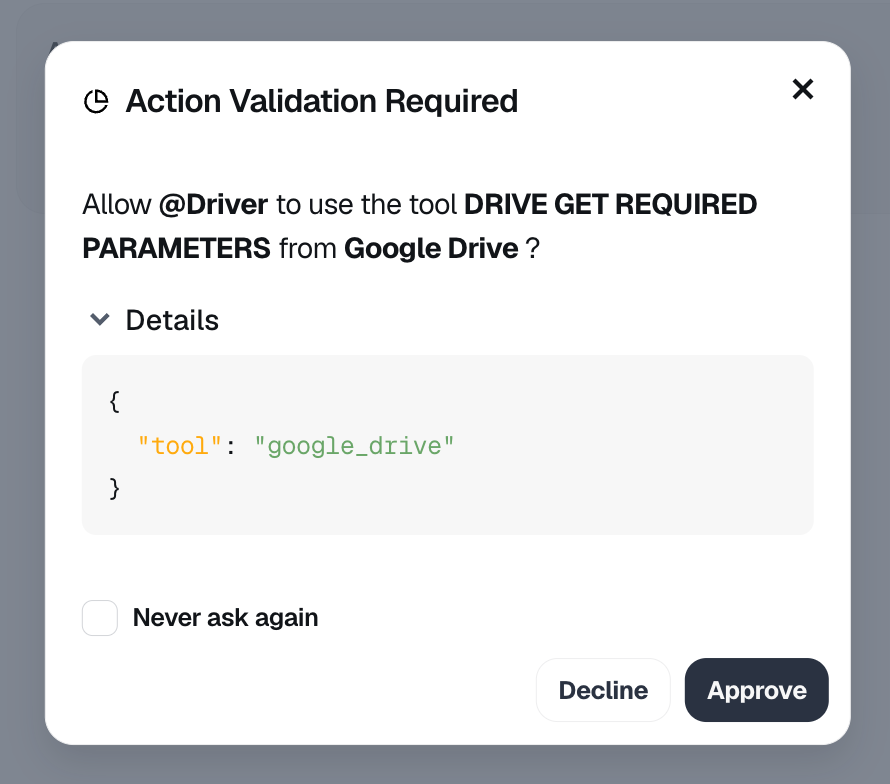
Example of a Low-stake validation pop-up.
Authentication
Remote Servers can, as the built-in toolsets, be added to several spaces, or available to everyone. They'll be available in the agent builder once they are added in a space you have access to.
Dust supports OAuth authentication for Remote MCP servers. When adding a Remote MCP server that requires authentication, the OAuth flow will automatically be triggered to set up the connection. This allows you to securely connect to MCP servers that implement authentication requirements.
Alternatively, you can add a Bearer token to your servers in Dust, that will be sent as an Authorization header in the calls made to it. You can edit this Bearer token by going to the Tools section of the Space tab.
Personal vs Workspace credentials
When adding a server that support OAuth authentication, you have the choice between Personal or Workspace credentials. Pick the one that is the most suitable for your use case.
- Workspace level credentials.
The authentication credentials you provide during setup will be shared by all users in this workspace when using these tools. - Personal level credentials.
Once setup for the workspace, each user will have to connect their own credentials to interact with these tools.
Pre-configuration
General introduction
When doing regular function calling the agent exposes to the LLM what parameters the function needs through a schema. The LLM then answers with valid parameters matching this schema, allowing the agent to effectively execute the function. References here and here.
This behavior integrates with the Model Context Protocol, more specifically the official TypeScript SDK, in the following way:
- When defining the tools for an MCP server a Zod schema the inputs of the tool will be validated against can be provided.
- When listing tools using the
tools/list
endpoint, this Zod schema is derived into a JSON schema, which is included in the response for the MCP client to show the LLM. - The answer of the LLM contains valid inputs, which can be passed in turn to the
tools/call
endpoint directly. When calling a tool the input is validated against the Zod schema.
All of this is abstracted when using the SDK, here is a minimal example for implementing an MCP server.
const server = new McpServer({
name: "my-server",
version: "1.0.0",
description: "Server description"
});
server.tool(
"tool-name",
"Tool description",
{
paramName: z.string().describe("Parameter description")
},
async (params) => {
// Tool implementation
}
);
Integration in the Assistant Builder
In some contexts, some of the parameters should be pre-configured. Notable examples include static URLs, mail addresses, certain inputs to API calls: parameters that are not known by the model and should be set statically when configuring the tool.
We provide input schemas that you can use when defining a tool to enable the following behavior:
- Input parameters can be defined and configured in the Assistant Builder.
- These parameters are hidden from the LLM.
- Pre-configured values are injected when the tool runs.
To leverage this feature, we expose in our TypeScript SDK reusable Zod schemas that you can add to your tool definitions to specify pre-configured inputs.
For instance, let's look at the following server definition.
import { INTERNAL_MIME_TYPES, ConfigurableToolInputSchemas } from "@dust-tt/client";
import { McpServer } from "@modelcontextprotocol/sdk/server/mcp.js";
import { z } from "zod";
const server = new McpServer({
name: "my-server",
version: "1.0.0",
description: "Server description"
});
server.tool(
"tool-name",
"Tool description",
{
query: z.string(), // Filled in by the LLM
name: ConfigurableToolInputSchemas[
INTERNAL_MIME_TYPES.TOOL_INPUT.STRING // Pre-configured
],
age: ConfigurableToolInputSchemas[
INTERNAL_MIME_TYPES.TOOL_INPUT.NUMBER // Pre-configured
],
hasApplied:
ConfigurableToolInputSchemas[
INTERNAL_MIME_TYPES.TOOL_INPUT.BOOLEAN // Pre-configured
],
},
async (params) => {
// Tool implementation
}
);
This server definition will lead to the following screen in the Agent Builder.
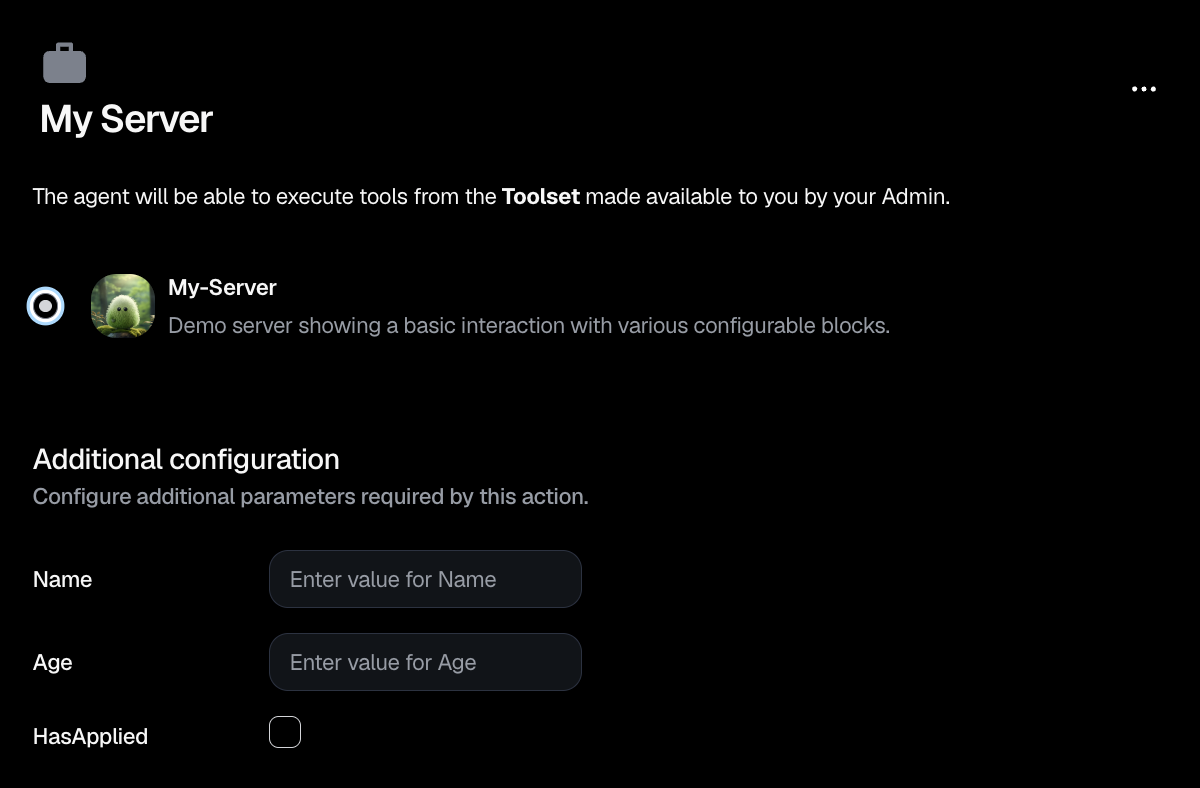
The configuration input here is tied to the agent configuration and will be returned as is when running the tool.
This mechanism in itself is language agnostic, if you are building MCP servers in another language, you have to define arguments in a way that will resolve to the same JSON Schema. For instance in Python you could use Pydantic in the following way:
from pydantic import BaseModel, Field
from typing import Literal
TOOL_INPUT_BOOLEAN_MIME_TYPE = "application/vnd.dust.tool_input.boolean"
TOOL_INPUT_NUMBER_MIME_TYPE = "application/vnd.dust.tool_input.number"
TOOL_INPUT_STRING_MIME_TYPE = "application/vnd.dust.tool_input.string"
class BooleanToolInput(BaseModel):
"""
Pydantic model for boolean tool input.
"""
value: bool
mimeType: Literal[TOOL_INPUT_BOOLEAN_MIME_TYPE]
class NumberToolInput(BaseModel):
"""
Pydantic model for numeric tool input.
"""
value: str
mimeType: Literal[TOOL_INPUT_NUMBER_MIME_TYPE]
class StringToolInput(BaseModel):
"""
Pydantic model for string tool input.
"""
value: str
mimeType: Literal[TOOL_INPUT_STRING_MIME_TYPE]
These will resolve into the following JSON Schemas:
{
type: "object",
properties: {
value: {
type: "boolean",
},
mimeType: {
type: "string",
const: "application/vnd.dust.tool_input.boolean",
},
},
required: ["value", "mimeType"],
}
{
type: "object",
properties: {
value: {
type: "number",
},
mimeType: {
type: "string",
const: "application/vnd.dust.tool_input.number",
},
},
required: ["value", "mimeType"]
}
{
type: "object",
properties: {
value: {
type: "string",
},
mimeType: {
type: "string",
const: "application/vnd.dust.tool_input.string",
},
},
required: ["value", "mimeType"]
}
Updated 3 days ago