This article explains how to build a Dust app that calls an external service using a cURL block. Specifically, it will call an API from https://open-meteo.com/ to get the current temperature, given coordinates.
The article then shows how to use that App from an Agent, and then how to use the Agent.
Creating the Dust App
Start by creating the App from the dashboard by going under Knowledge / Apps and clicking the New App button. Name it get_temperature, and use Retrieves the current temperature based on coordinates for the description. Then click Save to create the App.
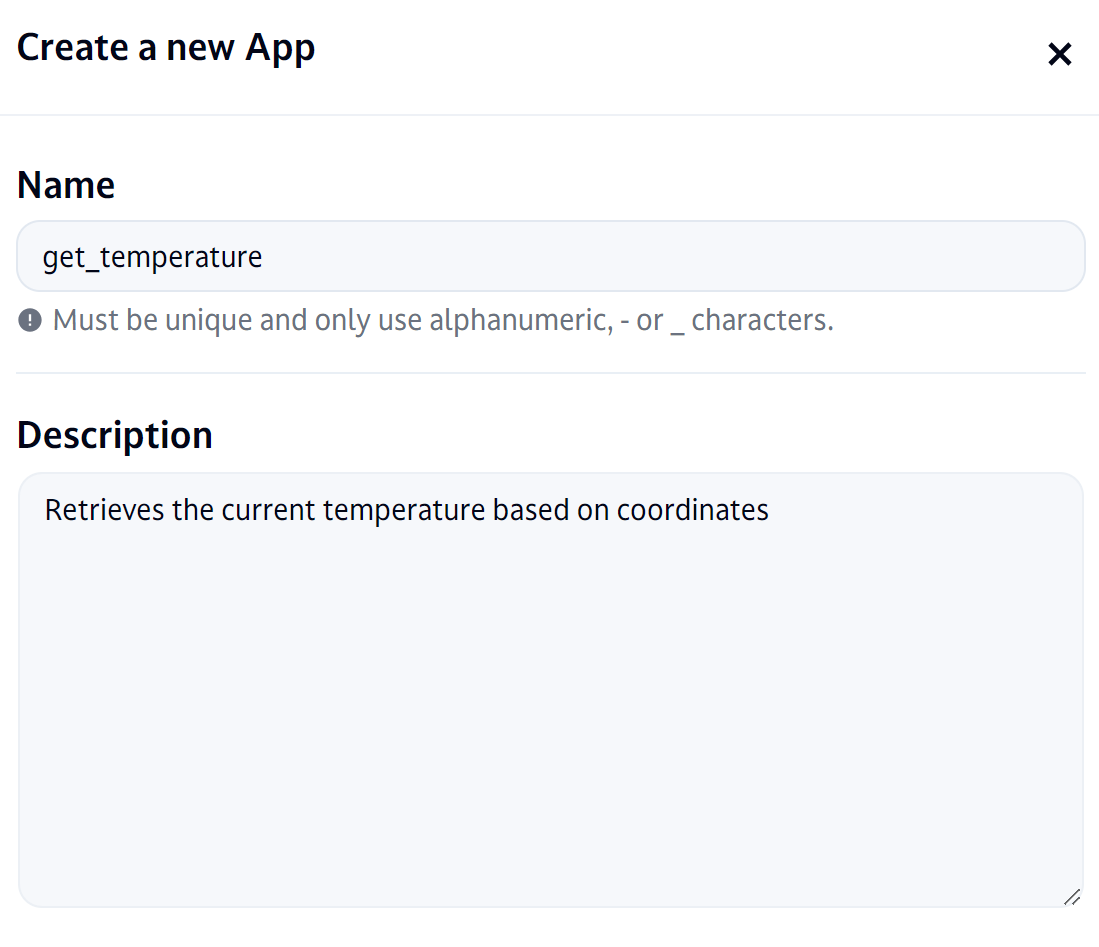
This App will be made of three Blocks:
- An
input
block that gets the latitude and longitude - A
curl
block that makes an http request to the external service to get the temperature - A
code
block that extracts the relevant part of the response, and returns it out
Creating the input block
Click Add Block, and create an input
block. Leave the block name as INPUT
, and click Create Dataset. Call it COORDINATES
, and optionally enter a description.
For the schema, create latitude
and longitude
fields, both of type number
.
For testing purposes, you should then enter a few pairs of coordinates in the data section. e.g. the ones below are for Paris and Seattle.
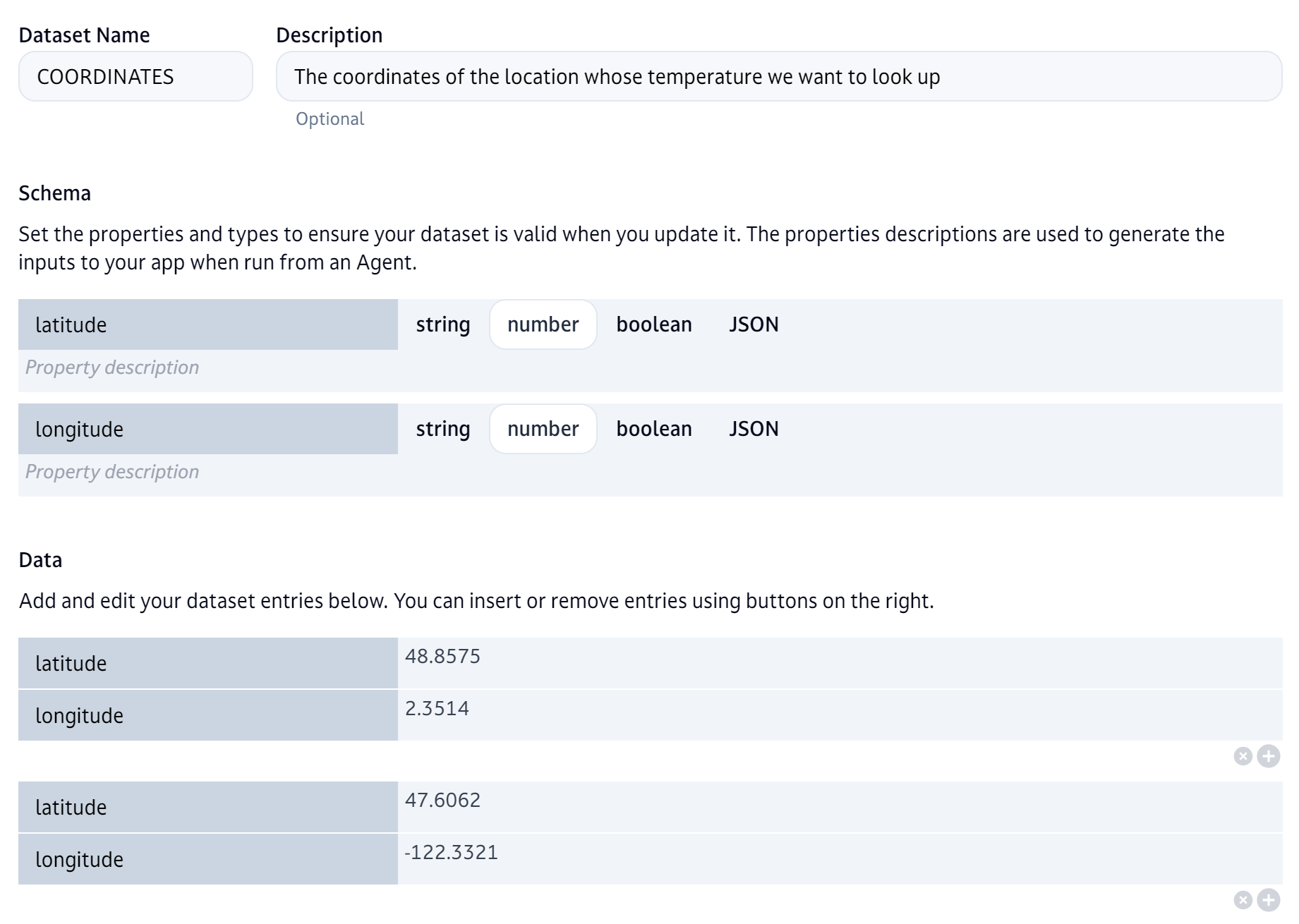
Click create to complete the dataset, and click on Specification
to see your blocks. In your input block, use the drop down to select your dataset.
Creating the curl block
Our next block will be used to actually send a request to the external API. Add a second block of type curl, and name it METEO
. Set the method to GET
, and set the URL to api.open-meteo.com/v1/forecast?latitude={{INPUT.latitude}}&longitude={{INPUT.longitude}}¤t=temperature_2m
. You can check out this page to see the docs of the forecast API we're calling.
The most interesting part is that we're getting the latitude and longitude from our INPUT
block, using the special {{INPUT.latitude}}
syntax to refer to the fields we defined in our input schema.
Change the Headers and Body code snippets to just return null. Since we're making a GET request, we don't need to pass a Content-Type
header, and GET requests don't have a body at all.
Here, even though we're not done, try clicking Run, which will let you observe what the output of the curl
block looks like. This will come useful in the next step.
Create the code block
Finally, let's create a 3rd block of type code
, which will be used to extract the temperature from the response of the request made by the curl
block. Call it EXTRACT_TEMP
, and use the following code:
\_fun = (env) => {
return env.state.METEO.body.current.temperature_2m;
}
Even if we're not familiar with the detailed output of this API, we can easily figure out that we want the body.current.temperature_2m
property by observing the output from the previous step.
You can now click Run again to try our sample coordinates through the entire app. Note that the external API won't actually be called this time, because the results are cached (you can disable that by clicking on the icon with the 3 stacked squares in the curl
block).
This is what your code
block should look like, along with the extracted temperatures (obviously, these will vary).
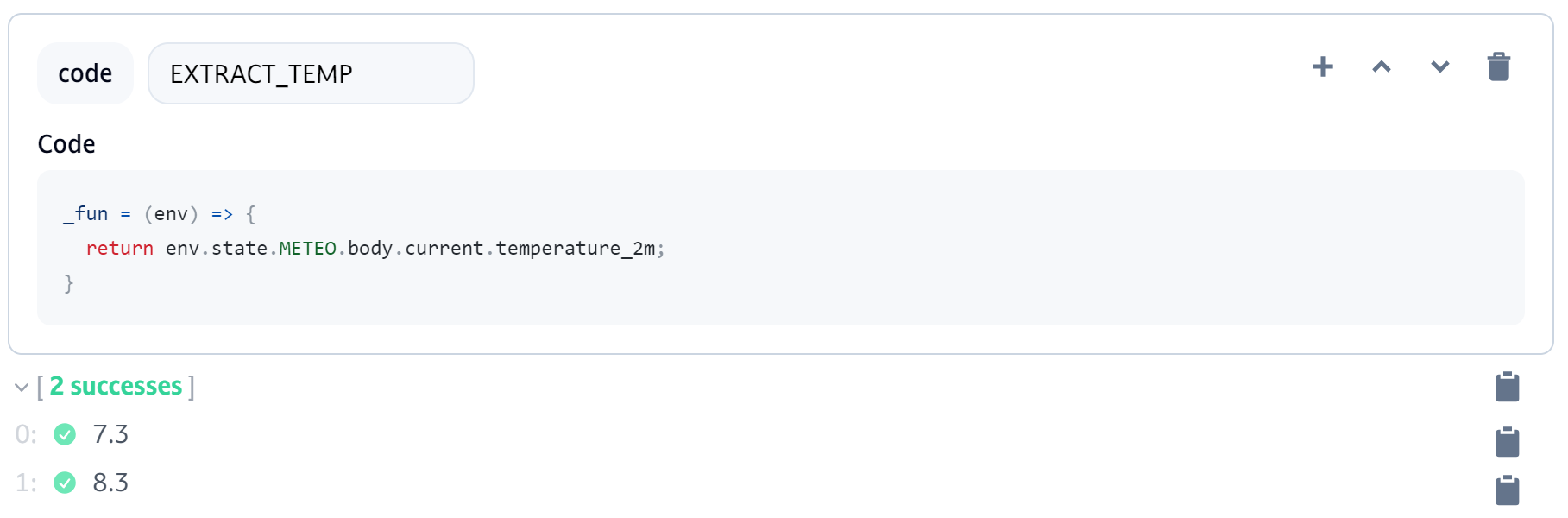
We're now done with our App!
Creating an Agent that uses our get_temperature App
In order to use an app in a chat, you first need to create an Agent that uses it. So let's now create such an Agent.
In the dashboard, under Chat, click ...
, and Create new agent
. Then click New Agent
to start from scratch. For the Agent instruction, enter the following:
Use the provided tool to retrieve the temperature for a given city. You will need to determine the cities coordinates before calling the tool.
Note that the second sentence is crucial, in order to tell the Model that given a place name, it should automatically figure out the coordinates. Without these instructions, the Model will prompt you, which is not what we want.
Click Next to go to the 'Tools & Data sources' step. Here, click 'Add a tool', and choose 'Run a Dust App'. You can then choose your get_temperature
app and click Save.
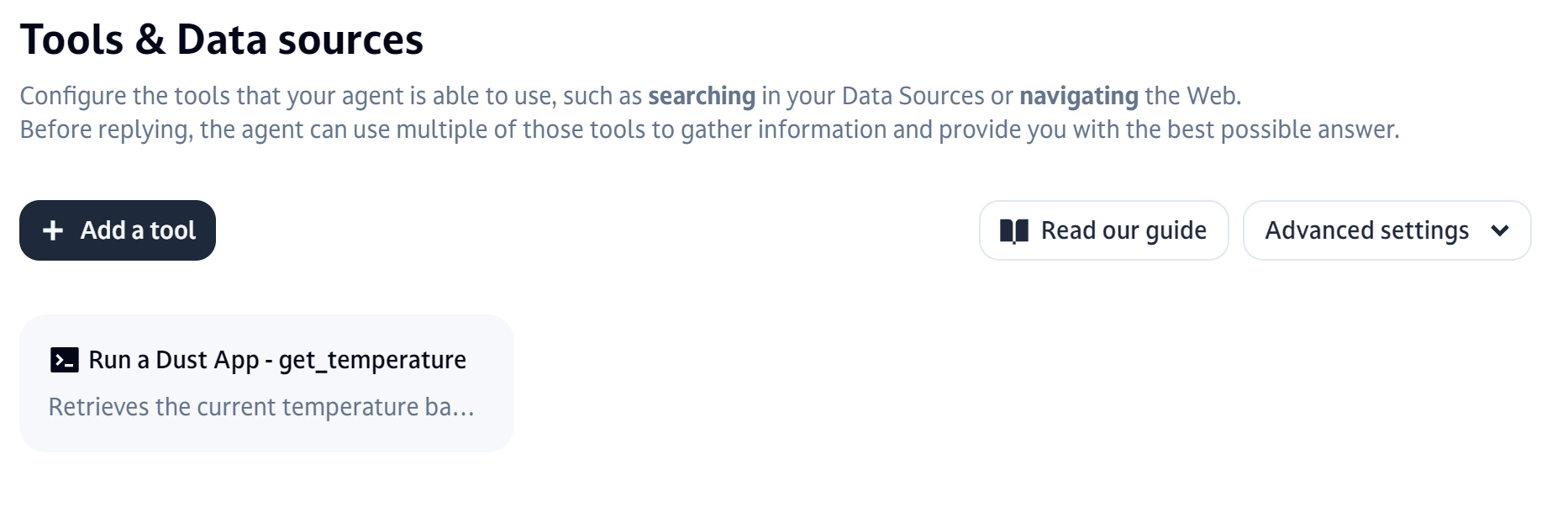
Click Next. In the naming step, give a handle to your Agent, e.g. @TempWizard
. Normally, the AI should have already generated a decent description, so you don't need to enter one.
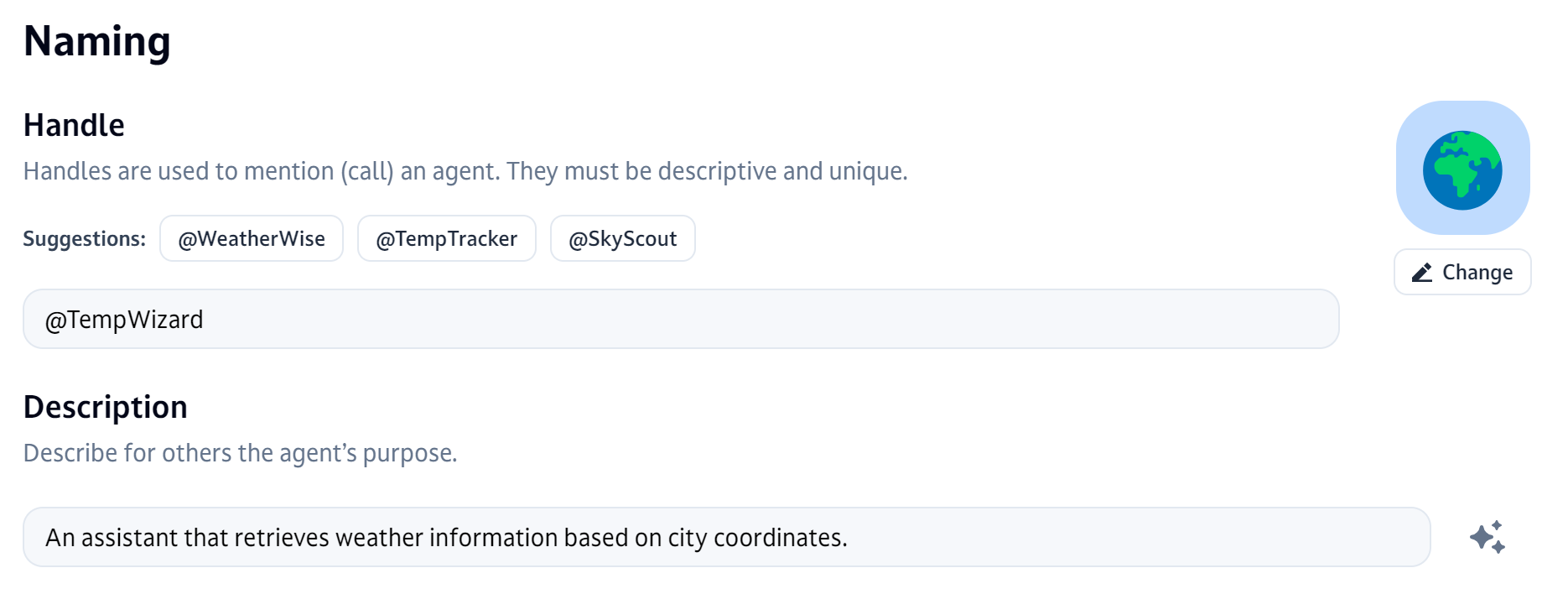
You can now click Save (upper right corner) to complete your Agent.
Using your agent
And now for the fun part, start a new chat, and ask a question to your new Agent, e.g. "@TempWizard what's the temperature in Sydney?"
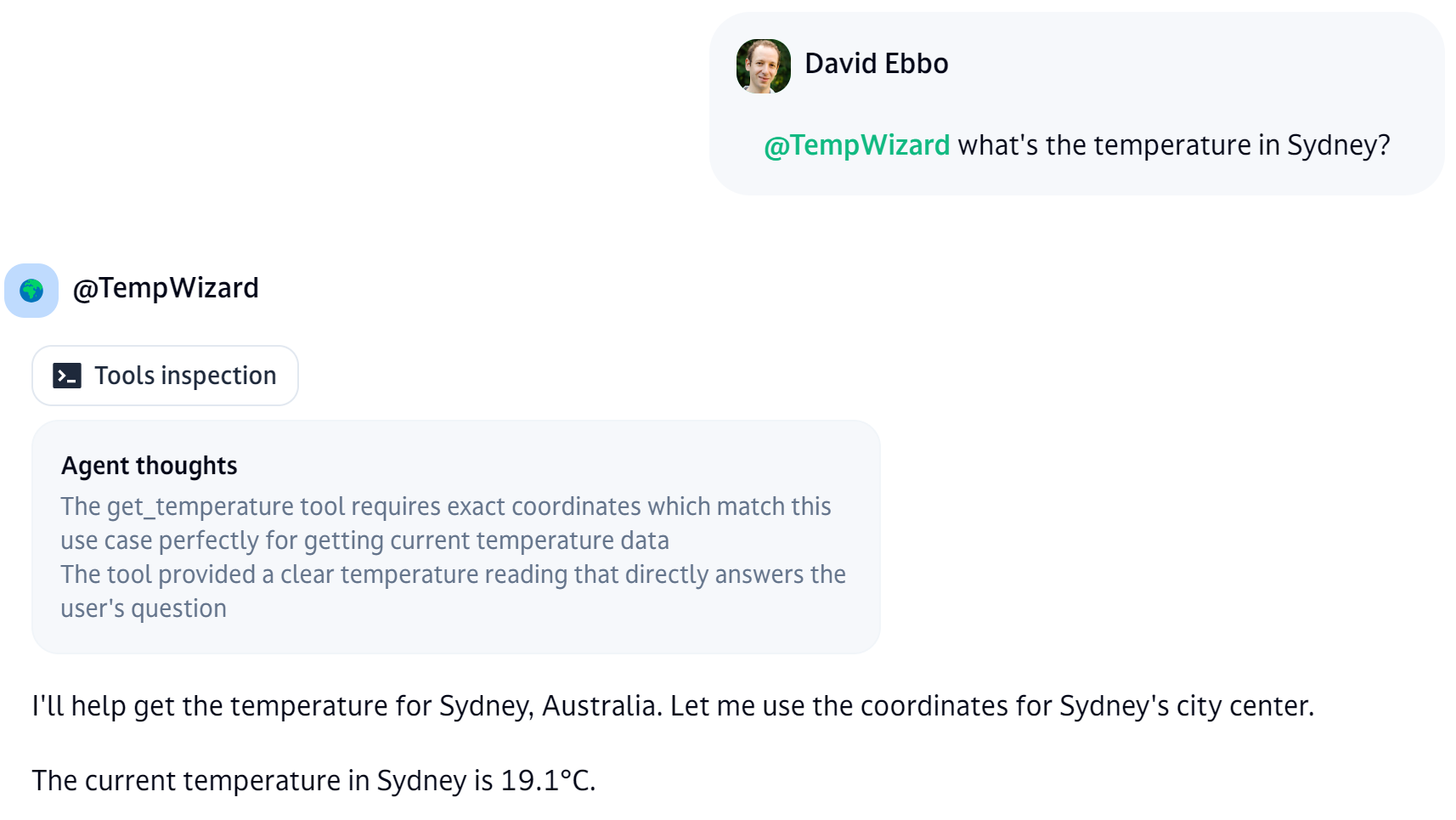
Read through the 'Agent thoughts', which helps understand how things work. The Model knows about your App, and sees that it's a good match to get a temperature. But it also sees that the App requires coordinates, while it only has a city. Thanks to the instructions we added to the Agent, it automatically gets the coordinates from the city before calling the App.